Published: Feb. 28, 2009, 9:57 p.m.
b'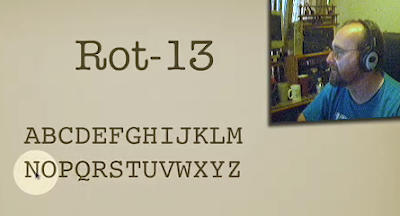
Starting Code:
import java.util.Scanner;
/**
* Simple Polyalphabetic Cryptography
*
* @Chris Thiel
* @28 Feb 2009
*/
public class VignereCipherStartingCode
{
private static final String alphabet="ABCDEFGHIJKLMNOPQRSTUVWXYZ";
private static int nextChar(String k, int i){
i=i%k.length();
String letter=k.substring(i,i+1);
return alphabet.indexOf(letter);
}
public static String encode (String m, String k){
String result="";
for (int i=0; i<m.length(); i++){
int offset=nextChar(k, i);
String letter = m.substring(i,i+1);
int newLetterIndex= alphabet.indexOf(letter)+offset;
newLetterIndex=newLetterIndex % 26;
result+=alphabet.substring( newLetterIndex, newLetterIndex+1);
}
return result;
}
public static String decode (String m, String k){
String result="";
return result;
}
public static void main(String[] args)
{
Scanner kb=new Scanner(System.in);
System.out.print("Type a key: ");
String key=kb.nextLine();
key=key.toUpperCase();
key=key.replace(" ","");//omit spaces
System.out.print("Type a message: ");
String message=kb.nextLine();
message=message.toUpperCase();
message=message.replace(" ","");
System.out.println("Using the key \\\\""+key+"\\\\" on \\\\""+message+"\\\\":");
String codedMessage=encode(message,key);
System.out.println("Coded="+codedMessage);
System.out.println("Decoded="+decode(codedMessage,key));
System.out.println("Using the key \'LOVE\' we encode \'BOOHOO\' we should get \'MCJLZC\' : "+encode("BOOHOO","LOVE"));
System.out.println("Using the key \'QUICK\' we decode \'TOKMXEQ\' we should get \'DUCKNOW\': "+decode("TOKMXEQ","QUICK"));
}
}
- 8 points: Complete the decode method so it can decipher the Vignere Cipher
- 9 points: Adapt this so it can do a Progressive Polyalphabetic Cipher rather than a key
- 10 points: Adapt the CarTalk Employee Applet\'s graphic user interface so that you can type a message in one text area, press a button and it shows the encoded text (using the Progressive Cipher), press another button and it shows the decoded text.
- 11 points: Add a "key field" to the Applet, and use the Vignere Cipher, working in a similiar fashion as the 10 point version
'